Often in game development, we find ourselves using multiple variables of same type. For example, when we need to create a set of materials to choose from, or set of colors to apply, or while setting random range values. We can also create individual variables for this purpose, but processing the data becomes more tedious. This is where arrays come in to the picture.
Arrays, allow you to group similar values into a single variable name and access them using index. In this tutorial, we will learn how to use arrays inside Unity with code examples.
Array in Unity
Arrays are fixed size collection of similar type variables. The maximum number of elements in an array is defined while initializing, and cannot be changed after that. But you can replace or remove an existing array data.
Array Initialization
To initialize an array, you need to specify the array data type followed by [] and name of the array. For example, let’s create an array of type integer.
int[] values;
Since, arrays have a fixed size, you need to specify the size of the array either while declaring or while initializing. Here is how to declare the size of an array.
int[] values=new int[5]; //array of size 5
if you want to assign the array size from the inspector window, then you can make the array public.
public int[] values;
And then, assign the array size in the inspector window.
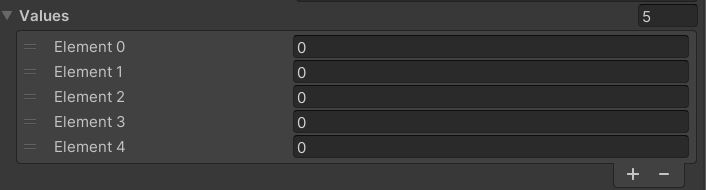
Assigning values to Array
To assign values to the array, you can either do it while declaring them or use the Start()
method.
Here is how to assign values while declaring in an array.
int[] values={1,2,3,4};
Or you can do it in the Start()
function like this.
int[] values;
void Start()
{
values=new int[]{1,2,3,4};
}
Or you can make the array public and assign the values in the inspector.
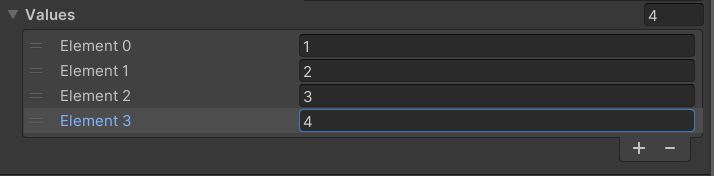
Operations with Array in Unity
A JavaScript array class, allows you to perform multiple operations like sort, add, remove and more. Unity does not allow all these functions inside a MonoBehaviour. You can use the Sort()
and Clear()
functions but you need to add the namespace using System
.
Now we will see all these operations one by one.
Displaying the array
We can use a for loop to run through the array and print the data one by one. Here is how to do it.
using UnityEngine;
public class ScoringSystem : MonoBehaviour
{
int[] values={4,2,3,1};
void Start()
{
for(int i=0;i<values.Length;i++)
{
Debug.Log(values[i]);
}
}
}
Sorting an Array in ascending order
We can use Array.Sort()
, which is a part of the System
namespace to arrange the array content incrementally. This also works on strings. Here is an example code.
using UnityEngine;
using System;
public class ScoringSystem : MonoBehaviour
{
int[] values={4,2,3,1};
void Start()
{
Array.Sort(values);
for(int i=0;i<values.Length;i++)
{
Debug.Log(values[i]);
}
}
}
Now let’s try this function on strings.
using UnityEngine;
using System;
public class ScoringSystem : MonoBehaviour
{
string[] values={"tommy","jonny","shek","itachi"};
void Start()
{
Array.Sort(values);
for(int i=0;i<values.Length;i++)
{
Debug.Log(values[i]);
}
}
}
And here is the output

Clearing an Array in Unity
We can clear a part of the array using the Clear()
function. Now let’s try to clear the values from index 1 to 3 in our array.
using UnityEngine;
using System;
public class ScoringSystem : MonoBehaviour
{
string[] values={“tommy”,”jonny”,”shek”,”itachi”};
void Start()
{
Array.Clear(values,1,3);
for(int i=0;i<values.Length;i++)
{
Debug.Log(values[i]);
}
}
}
The output of the above code prints null for all the index range specified in the Clear()
function.

2D Array in Unity
Unity also allows users to create multi-dimensional array. Depending on the number of dimensions you want in an array, you can add that many array sizes separated by comma. Here is an example of a 2D array.
string[,] values=new string[2,4]{{"tommy","jonny","shek","itachi"},{"English","French","Hindi","Japanese"}};
You can think of a 2D array as a 2D matrix. The length of each column must be the same and length each row must be the same.
Here is how the above array can be visualized.

To access the string “jonny”, you can use the row and column index value like this.
using UnityEngine;
public class ScoringSystem : MonoBehaviour
{
string[,] values=new string[2,4]{{"tommy","jonny","shek","itachi"},{"English","French","Hindi","Japanese"}};
void Start()
{
Debug.Log(values[0,1]);
}
}
You can print all the values in the 2D array using nested for loop. You can use GetLength(0)
and GetLength(1)
to get the row and column length of a 2D array. Here is the code sample to print the complete 2D array.
using UnityEngine;
public class ScoringSystem : MonoBehaviour
{
string[,] values=new string[2,4]{{"tommy","jonny","shek","itachi"},{"English","French","Hindi","Japanese"}};
void Start()
{
for(int i=0;i<values.GetLength(0);i++)
{
for(int j=0;j<values.GetLength(1);j++)
{
Debug.Log(values[i,j]);
}
}
}
}
Array of arrays
An array of arrays is essentially an array where each element is another array. Each inner array can have a different length, allowing for more flexibility in representing irregular grids.
To declare an array of arrays you have to use two square brackets as shown in the code sample below.
string[][] values={new string[]{"tommy","jonny","shek","itachi"},new string[]{"English","French"}};
Array of arrays is different than the 2D array and can be represented by just one index. For example, values.Length
will give you the number of arrays stored and values[0].Length
will give you the length of the array at index 0.
To print the complete arrays, we can use a nested for loop we did with the 2D arrays. Here is the code sample for that.
using UnityEngine;
public class ScoringSystem : MonoBehaviour
{
string[][] values={new string[]{"tommy","jonny","shek","itachi"},new string[]{"English","French"}};
void Start()
{
for(int i=0;i<values.Length;i++)
{
for(int j=0;j<values[i].Length;j++)
{
Debug.Log(values[i][j]);
}
}
}
}
Hope this gives you a clear idea about arrays in Unity. If you have any other questions, feel free to leave them in the comment box below.