Unity SphereCast function casts a sphere in the specified direction to the specified distance, and returns any object that was hit along the path. SphereCast works in a very similar way to Unity Raycast the only difference being the sphere which is cast in place of a ray.
Unity SphereCast Syntax
public static bool SphereCast(Vector3 startPoint, float radius, Vector3 direction, out RaycastHit hit, float maxDistance = Mathf.Infinity, int layerMask = DefaultRaycastLayers, QueryTriggerInteraction queryTriggerInteraction = QueryTriggerInteraction.UseGlobal);
Input Number | Function |
---|---|
1 | Starting point of the Sphere. |
2 | Radius of Sphere |
3 | Direction of SphereCast |
4 | Output Hit data variable |
5 | Maximum distance of Cast |
6 | Layer ignore or include details |
7 | Whether to hit trigger colliders or not |
Unity SphereCast Script Demo
SphereCast Script
using UnityEngine;
public class PlayerCast : MonoBehaviour
{
public float radius;
public float maxDistance;
public LayerMask layerMask;
RaycastHit hit;
// Update is called once per frame
void Update()
{
if(Input.GetKeyDown(KeyCode.Space))
{
Cast();
}
}
void OnDrawGizmos()
{
Gizmos.color=Color.red;
Gizmos.DrawSphere(transform.position-transform.up*maxDistance,radius);
}
void Cast()
{
if(Physics.SphereCast(transform.position,radius,-transform.up,out hit,maxDistance,~layerMask))
{
Debug.Log(hit.collider.gameObject);
}
}
The above script casts a ray in the downward direction from the object to which the script is attached. The user can specify the radius of the sphere and the max distance to which the ray is to be cast.
OnDrawGizmo function is used to visualize the SphereCast in the scene view. A sphere of size of the specified radius will be drawn at the max distance value.
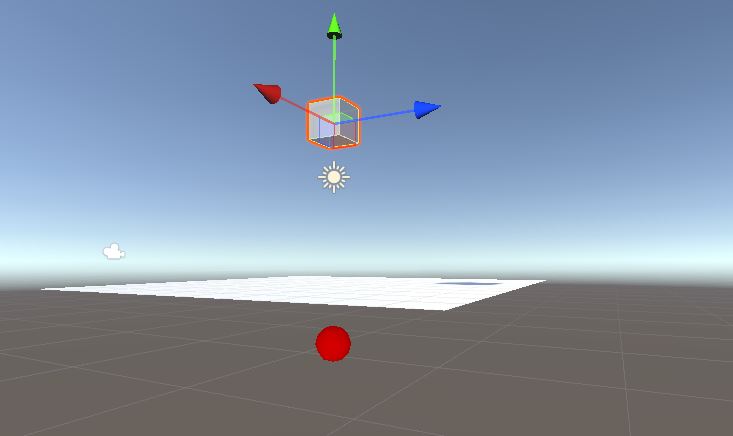
Optional parameters of Unity SphereCast
Not all parameters are to be specified to successfully cast a sphere. You just need to specify the start point, radius and direction. All the other parameters will be set to default.
Default values
- No output data, returns true if SphereCast hits a collider
- Distance is set to infinite by default
- Layer- Includes all layer
- QueryTriggerInteraction is set to project settings by default.
Reasons for SphereCast not working
SphereCast returns true only if it hits a collider. Here are some things to check if your SphereCast is not working
- Check if the sphere radius is too big. If the sphere is touching the target object at the starting point, then it will ignore the collider.
- Check if the sphere radius is too small. If it’s too small it might miss the object.
- Check if you are casting the sphere in the right direction.
- Check layer details. You might be ignoring the layer.
It’s a good practice to use a gizmo to know if you are casting the sphere in the right direction as in the above code sample.
SphereCastAll
A regular SphereCast returns the first object that it has hit. But if you need all the objects in the direction specified then you have to use SphereCastAll.
The syntax of SphereCastAll is little different than SphereCast. Here is an example script to understand it.
using UnityEngine;
public class PlayerCast : MonoBehaviour
{
public float radius;
public float maxDistance;
public LayerMask layerMask;
RaycastHit[] hits; //SphereCastAll outputs an Array
// Update is called once per frame
void Update()
{
if(Input.GetKeyDown(KeyCode.Space))
{
Cast();
}
}
void OnDrawGizmos()
{
Gizmos.color=Color.red;
Gizmos.DrawSphere(transform.position-transform.up*maxDistance,radius);
}
void Cast()
{
hits=Physics.SphereCastAll(transform.position,radius,-transform.up,maxDistance,~layerMask);
Debug.Log(hits.Length);
}
}
SphereCastAll outputs an array of object and unlike SphereCast, it includes the object from which it originates.
The above code will get all the object in the downward direction and output the number of objects hit.
Conclusion
In conclusion, Unity SphereCast provides a powerful alternative to the traditional Raycast method in Unity game development. With its ability to detect collisions with complex and irregular shapes, SphereCast offers a more accurate and efficient solution for detecting object intersections.
Additionally, SphereCast provides the ability to specify a radius for the cast, which can help to simulate more realistic physics in game environments. While Raycast still has its uses, particularly for simple straight-line collisions, SphereCast is the superior method when dealing with more complex geometry.