Unity is a feature rich game engine and there is no one definite way to do anything. You can come up with your own logical way to make your game. But learning some Unity tips can help you optimize the workflow and game performance. In this article, we will see the top 5 tips that beginners can use while making their game.
1. Initialize function when scene is loaded
Similar to how Awake and Start are called on scene load, you can create your own custom function that is called when a scene is loaded. These functions are called after Awake function is called. These methods should be set as static while declaring them.
In the below example script, we create a class which is not derived from MonoBehaviour and make the functions of the class initialize on scene load without user calling them.
using UnityEngine;
class CustomClass
{
[RuntimeInitializeOnLoadMethod]
static void MyMethod()
{
Debug.Log("After Scene is loaded and game is running");
}
}
You can also initialize the method before scene load using a parameter as shown below. In this case the function is called before the Awake function.
using UnityEngine;
class CustomClass
{
[RuntimeInitializeOnLoadMethod(RuntimeInitializeLoadType.BeforeSceneLoad)]
static void MyMethod()
{
Debug.Log("After Scene is loaded and game is running");
}
}
2. Onclick Event to Disable a component
When you need to disable a component of a gameobject on UI button press, the common practice followed by beginners is to create a public function, link it to the onClick event of the button, get the game object, get the component and then disable it.
There is a shorter way to do it. You can directly drag and drop the gameobject on to the Onclick event and disable the component without a script as shown in the image below. You can also do this for a prefab.

3. Inspector Debug Mode
When you need to check the value of a non-serialized or private variable during runtime, the common practice is to use the debug log and display the variable value on the console window.
This is not required as you can use the inspector debug mode to check the values of all variables in your script. To put the inspector in debug mode, click on the three dots on the top and select Debug. You can switch back to normal mode by selecting the normal mode.
You can also open up, another inspector window and make one as debug window and the other as normal window.
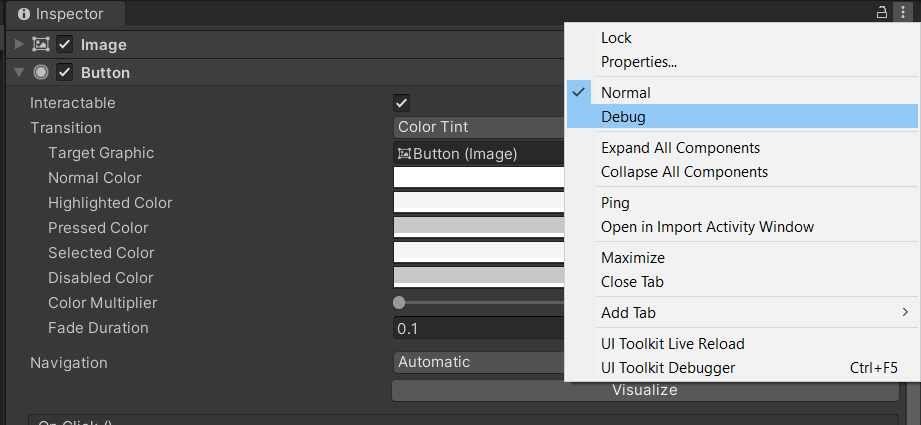
4. Lock Inspector window
Have you ever tried to drag a material to a gameobject’s property in the inspector window, only to find that the inspector property has changed to the material? The solution is locking your inspector. There are two ways to do it.
First method is to lock the existing inspector by clicking on the lock icon on the top of the inspector window.
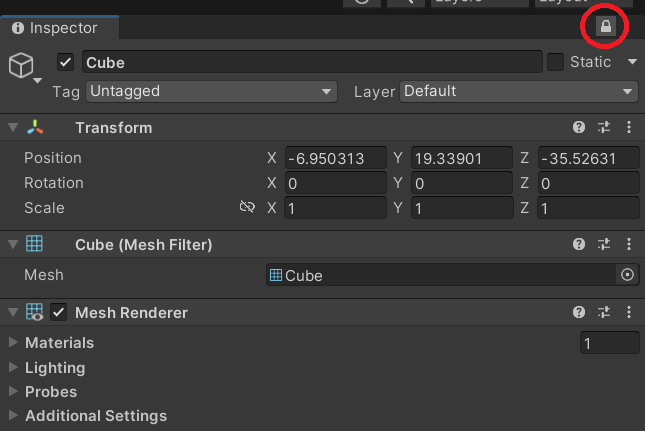
The second method is to open a custom inspector that displays only the properties of the gameobject. You can right click on the game object and select properties.
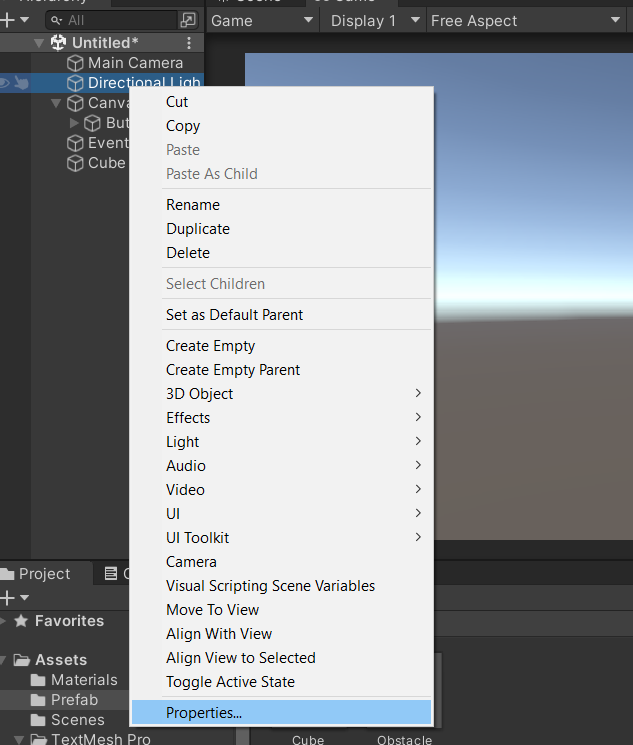
5. Instantiating a Component
In most of the cases, we need to get a component of the instantiated object. The general practice is to instantiate the gameobject and assign it to another variable. Then use the GetComponent method to get the component.

Did you know? Unity instantiate takes in a component as input and instantiates the gameobject while assigning the component to the variable. This reduces the unnecessary GetComponent calls and improves performance. Here is the optimized code
using UnityEngine;
public class SpawnProjectile : MonoBehaviour
{
public Rigidbody projectile;
Rigidbody spawnedProjectile;
float forceMagnitude=5;
void Start()
{
spawnedProjectile=Instantiate(projectile,transform.position,Quaternion.identity);
spawnedProjectile.AddForce(transform.forward*forceMagnitude,ForceMode.Impulse);
}
}
Hope these tips will be useful on your game development journey.