Creating a Car controller in Unity is different than regular character controllers. While moving a character we try to move the whole body and add animations to the character to make it look like its moving. But in case of a car, we use wheel collider physics to move the car and update the wheel rotation based on that. It might sound complex but it’s not. You will understand it once you have created your first Unity car controller.
I am using Unity 2021.3.11 for this tutorial. But not much has changed when it comes to wheel collider physics in Unity. So, it doesn’t matter which version of Unity you are using, this tutorial should hold good.
Video Tutorial
Getting your scene ready
The first thing that you will need to create a car controller is a car. So, if you don’t have one, you can download a car from Unity asset store.
For the purpose of this tutorial, I am using the Cartoon Low Poly City Pack Lite free asset from the asset store. and I am going to load up the demo scene from the asset.
It’s up to you on which asset you use, but make sure the car’s wheels can be rotated separately from the body.
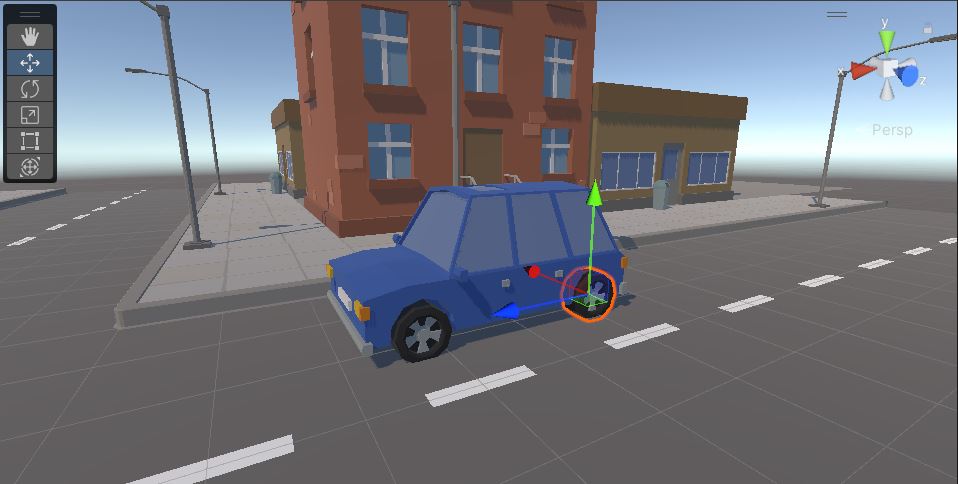
Getting the Car ready
This is a very important step when creating a car controller. You cannot just add a wheel collider to all car wheels and start rotating them. Unity requires you to follow a certain standard on how the Car wheels and wheel colliders are arranged inside the mesh.
The main parent car should have a Rigidbody component attached to it. Set the mass to 1500 which will act as the weight of the car.
Unity requires you to arrange all the wheel inside a parent game object and all the Wheel Colliders in a separate parent game object. Take a look at the picture below to see how the car prefab has been rearranged.

The position of the Wheel and the Wheel collider should be the same. That is if Wheel_01 is at 0,0,0 then Wheel_Collider_01 should also be at 0,0,0. The Wheel Collider game object should only have a transform and a wheel collider component attached to it. Also, there should be no other collider attached to the Wheels.
This step is not required but your car won’t look like its moving on the wheels if you don’t position them correctly.
Wheel Collider is Not visible
After the last step you should see the Wheel colliders around your wheel. If you don’t then check the following.
- Did you add a Rigidbody to the parent car gameobject. (Make sure you didn’t add the Rigidbody to the body part)
- Enable Wheel collide Gizmo in the scene view.
Car shaking or jumping automatically after adding Wheel Colliders
If you are car is jumping around automatically then it’s because of the interaction between the Wheel Collider and the car bodies mesh collider. Replace the mesh collider with a box collider and make sure than the box collider is not intersecting with the Wheel Colliders.
Also check if any of your wheels or other car parts have other colliders attached to it.

Car Controller Script
Now we are all set to drive our car. It time to get that driving script ready. Before we dive into the coding part you need to know some basics of driving with Wheel Colliders.
Motor torque
Motor Torque can be applied to individual wheel colliders to move the wheels forward or backward. It’s measured in Nm and you need to apply the torque based on the mass and friction forces acting on the car.
Steer Angle
This is the angle by which the wheels need to rotate to turn your car. You can set the Steer angle to the degrees to which you want the car to turn. Remember to clamp this value to a max value or you might have some unwanted results.
Brake torque
Reverse Motor torque can be applied to stop the car but the time taken is more and the car stops more slowly than expected. To achieve instant breaking, we can apply a large brake force
We are going to set the steer angle when the player operates the left and right arrow key and we will set the motor toque when the player operates the Up and down arrow keys. We will set the steering angle and motor torque only to the front wheels.
We will be using Fixed update since its all physics.
using UnityEngine;
public class car_controller : MonoBehaviour
{
public WheelCollider[] wheel_col;
public Transform[] wheels;
float torque=100;
float angle=45;
void Update()
{
for(int i=0;i<wheel_col.Length;i++)
{
wheel_col[i].motorTorque=Input.GetAxis("Vertical")*torque;
if(i==0||i==2)
{
wheel_col[i].steerAngle=Input.GetAxis("Horizontal")*angle;
}
var pos=transform.position;
var rot=transform.rotation;
wheel_col[i].GetWorldPose(out pos,out rot);
wheels[i].position=pos;
wheels[i].rotation=rot;
}
if(Input.anyKeyDown)
{
if(Input.GetKeyDown(KeyCode.Space))
{
foreach(var i in wheel_col)
{
i.brakeTorque=2000;
}
}
else{ //reset the brake torque when another key is pressed
foreach(var i in wheel_col)
{
i.brakeTorque=0;
}
}
}
}
}
Final Output
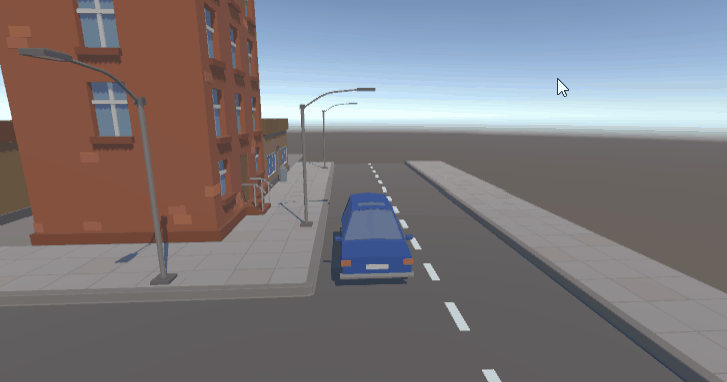