In this tutorial, we will see how to drag and drop an UI icon into a slot using the UI event system in Unity.
We will be using the IDragHandler and IDropHandler of the event system for this purpose. The IDragHandler has a Begin,Drag and an End method that we can use to control the object being dragged. The IDropHandler has the OnDrop function that we can use to fit the object into the slot.
We will also adjust the transparency of the sprite when its being dragged and set it back to normal when its placed in the slot.
Video Tutorial
Setting up the Sprites
I have two sprites. One Axe which will be our object and the other is for the slot.
The texture type of both the sprites needs to be set as 2D and UI. Create two 2D UI image. One for the Object and the other for the slot.
Drag and drop the sprites to the images. Remember to place the object last in the order after the slot. Otherwise, the object will be hidden behind the slot.
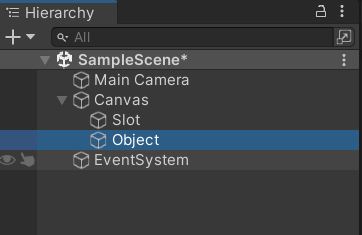
The Drag Script
Let’s add the Drag script to the Object. We will set the alpha value of the object to 0.5 when the drag begins and set it back when the drag ends. During the drag we will add the delta moved the mouse to the position of the object. The delta value is available in the event data. We need to adjust the delta value to the scale of the canvas. So, we can just divide the delta by Canvas scale factor.
Create a Script called DragScript and add the code below and assign the canvas in the inspector.
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.UI;
public class DragScript : MonoBehaviour,IDragHandler,IBeginDragHandler,IEndDragHandler
{
RectTransform rec;
Image img;
Color tempColor;
[SerializeField] Canvas canvas;
void IBeginDragHandler.OnBeginDrag(PointerEventData eventData)
{
tempColor.a=.2f;
img.color=tempColor;
}
void IDragHandler.OnDrag(PointerEventData eventData)
{
rec.anchoredPosition+=eventData.delta/canvas.scaleFactor;
img.raycastTarget=false;
}
void IEndDragHandler.OnEndDrag(PointerEventData eventData)
{
tempColor.a=1;
img.color=tempColor;
img.raycastTarget=true;
}
// Start is called before the first frame update
void Awake()
{
rec=GetComponent<RectTransform>();
img=GetComponent<Image>();
tempColor=img.color;
}
}
The Drop Script
When we end the drag the object is left in the same position when the drag ends. But we need it to fit exactly into the slot. For that we can use the Drop handler to set the position of the object to the position of the slot if the drag has ended on the slot.
We need to uncheck Raycast target on the object so that the OnDrop function is called on the slot. This is already taken care in the OnDrag function in the script above. Create a new DropScript and add the code below to adjust the position to the slot on drag end.
using UnityEngine;
using UnityEngine.EventSystems;
public class DropScript : MonoBehaviour,IDropHandler
{
void IDropHandler.OnDrop(PointerEventData eventData)
{
eventData.pointerDrag.GetComponent<RectTransform>().anchoredPosition=GetComponent<RectTransform>().anchoredPosition;
}
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
}
Final Result
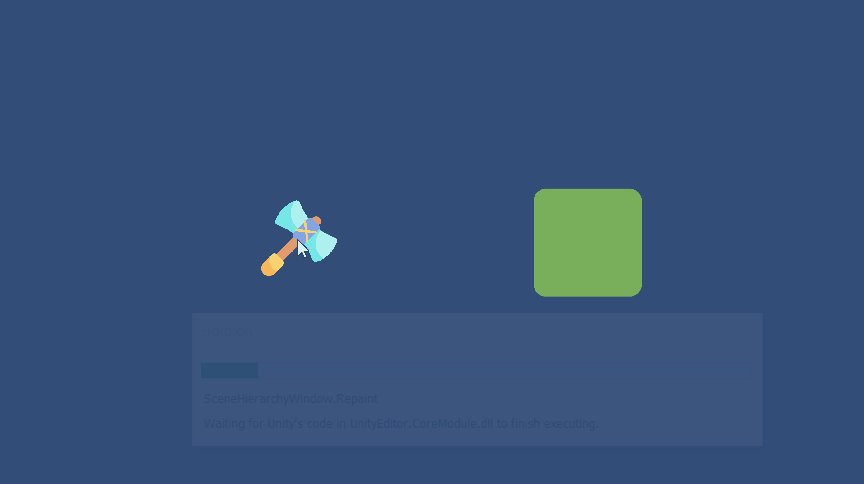