Games contain tons of scripts and there is always a need to access data from another script. Be it player health or for scoring system, you need to access another script to either display the value or to modify it. In this tutorial, we will see how to access a variable from another script in Unity.
Before we jump into the code, let’s understand the basics.
Scope of Variables
To access a variable or a function from another script, you need to set it as public.
Here is an example of a variable scope set to public.
public int Score;
If you declare a variable without the scope like int Score;
then its consider as private by default and cannot be accessed from outside the class.
Also, all the variables declared inside a loop or a function, can only be accessed from within the loop or function.
Object of a class
To access a class variable or a method, you need an object of the class. Think of the class as a software hosted online. In order to use the software, you need a login. This login is nothing but a key to hold a customized version of the software with your personal data. Depending on what login you use, the data you see inside will vary.
Similarly, to access the class features, we need to create an object of the class. Here is an example of creating an object for the class “ScoringSystem” in C#.
ScoringSystem score = new ScoringSystem();
Using the above statement will call the constructor of the class. Since, most of the scripts in Unity are inherited from MonoBehaviour and do not have a constructor, using the new keyword will give you a warning as shown in the image below.
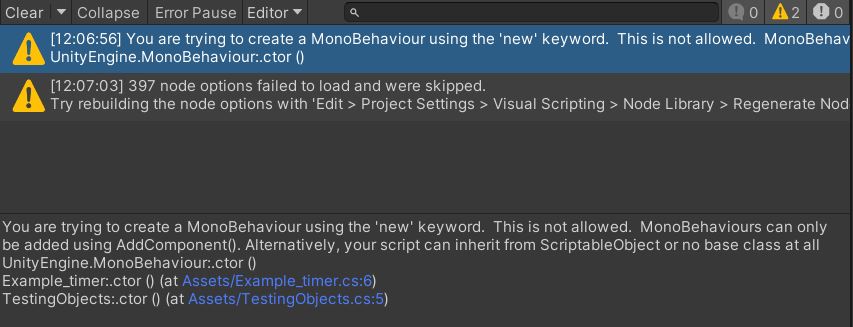
The reason for this is Unity requires an object reference. So, let’s try to understand that next.
Understanding game object reference in Unity
In a game, a single script can be used by multiple game objects. In order to know, which script we are trying to access, Unity needs us to specify the object to which the script is attached.
There are two ways to do it. One is using GetComponent
function and the other is by specifying the object in the inspector.
Taking the example of the scoring system, let’s say the script is attached to a Player game object. We use GameObject.Find
to find the player and then use GetComponent
to get the script attached to it.
Here is how you need to use it.
ScoringSystem my_object;
void Start()
{
my_object=GameObject.Find("Player").GetComponent<ScoringSystem>();
}
The second way, is to declare the object as public.
public ScoringSystem my_object;
Now, you will be able to assign the game object in the inspector as shown in the image below
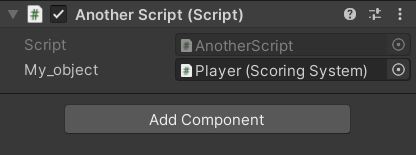
Accessing Variable from another script
Now that we understand all the concepts, let’s demo it with an example. Say there is a player game object with a scoring system script attached to it. The script has a public variable score. Let’s create another script and attach it to an empty game object. This object will log the score value when game starts.
Here is the scoring system script.
using UnityEngine;
public class ScoringSystem : MonoBehaviour
{
public int score=20;
}
This is the other script that will log the score value to the console.
using UnityEngine;
public class AnotherScript : MonoBehaviour
{
ScoringSystem my_object; //declaring object of the class
void Start()
{
my_object=GameObject.Find("Player").GetComponent<ScoringSystem>(); //assigning the object reference
Debug.Log(my_object.score);
}
}
Using GameObject.Find
can be performance expensive if used many times. So, the other option is to change the scope of the class object to public. You will need to assign the game object in the inspector in this case.
Here is the code for that
using UnityEngine;
public class AnotherScript : MonoBehaviour
{
public ScoringSystem my_object;
void Start()
{
Debug.Log(my_object.score);
}
}
Hope all the concepts are clear to you. If not, feel free to leave a comment below. You can access static variable without an object. To know more, read our tutorial on Singleton and static variables.