The complete introduction to Unity game development
Here's how to get started with the Unity game engine, from installation to familiarizing yourself with the interface and options.
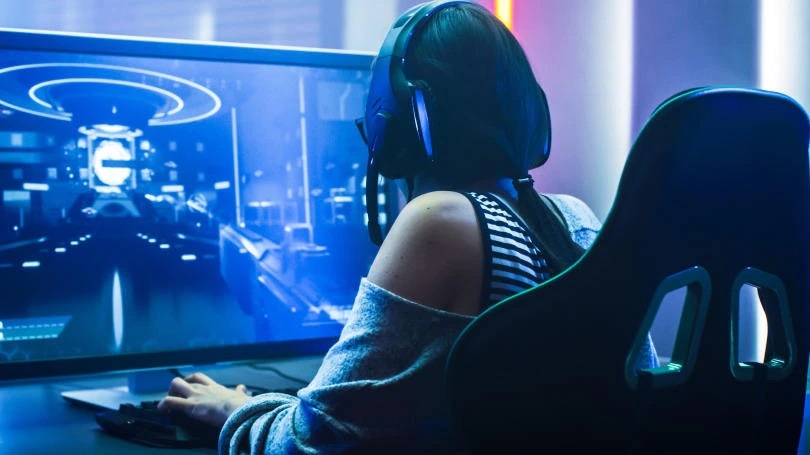
Stepping into the world of game development with Unity can feel like being handed the controls to a spaceship. The interface is full of buttons, sliders, and panels, each with its own specific purpose. It's easy to feel overwhelmed, especially if you're new to game development. However, once you learn the essentials, this interface will transform from a daunting science-fiction control panel to a powerful canvas for your creativity.
Table of contents:
Installing Unity
Unity can be installed directly, but it is more common for developers to manage installation of one or more versions via the Unity Hub—a program that can download and automatically update any number of simultaneous installation of Unity, and which manages user-level software licenses and preferences. Unity Hub can be downloaded from https://unity.com/download by selecting the appropriate download for your operating system (Windows, Mac, or Linux). Once Unity Hub is installed, a specific Unity Editor version can be installed via the Installs page by selecting "Install Editor." If you have no specific constraints about which version to install (for example, if you relied on a framework that only worked with a specific version), just pick the latest version with LTS (long-term support, which means Unity has committed to maintain this version for a long time).
Note that if you have never used Unity before it may require you to register a license by providing an email address and agreeing to Terms of Service. In doing this, you agree that by using the free version of Unity you are not using it for certain purposes—such as to earn over some specified amount of income each year, typically in the hundreds of thousands of dollars—and that if you are, you will instead convert to a paid subscription.
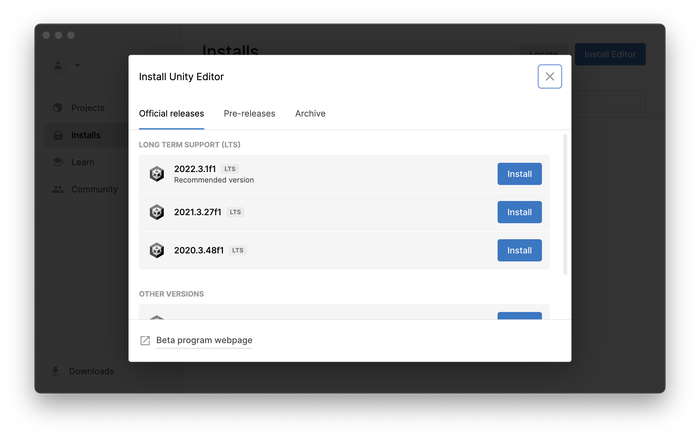
Figure 1: Selecting a Unity Editor version to install.
Creating Your First Project
Creating your first project just means selecting Projects - "New project" and choosing a template to start from. Here, templates are available which determine which frameworks will be installed by default when the project opens. For most purposes, picking just "2D" or "3D" is perfectly fine. Select where to save your project and what to call it, but these can always be changed later.
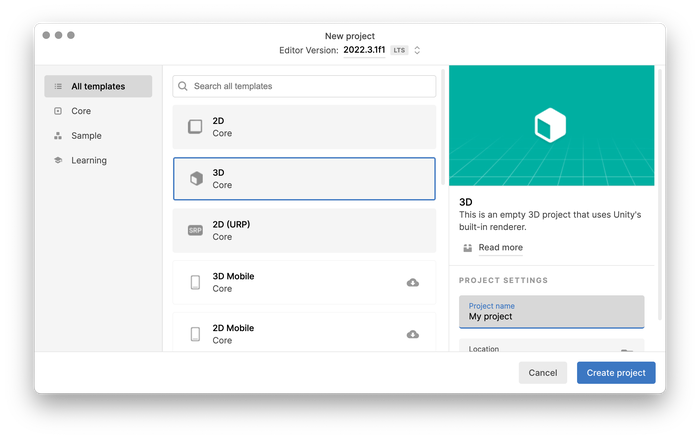
Figure 2: Creating a new project in Unity.
The User Interface
The Unity Editor interface is designed around the concept of flexibility. It's modular—meaning you can rearrange the layout to suit your preferences. But let's start with the default layout. In this, the interface is divided into five main sections: the Scene view, Hierarchy, Inspector, Project, and Game view. Dominating the center of your screen, the Scene view is where you'll spend a significant amount of time. This is where you manipulate your game objects in a 3D or 2D space, moving them around, scaling them, or rotating them to create your game world.
The concept of a "Scene" in Unity is also really important: games in Unity are structured from multiple scenes. Scenes also exist as a file inside your project's folder, and a game will typically transition among multiple scenes in various ways. For example, your game's menu will be a scene, but also each level might be a scene, a loading screen will be a scene, and so on.
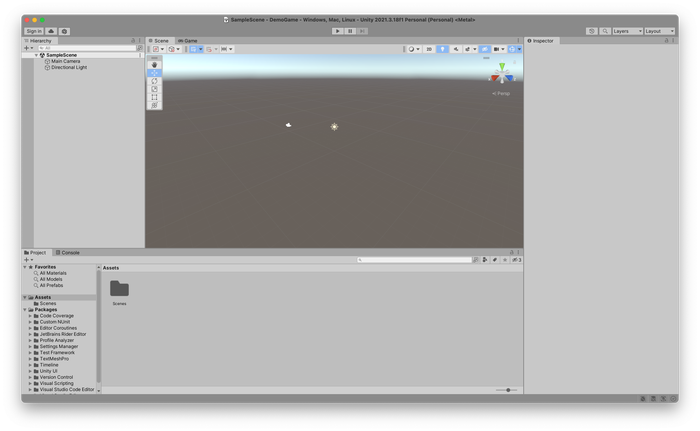
Figure 3: The Unity Editor interface
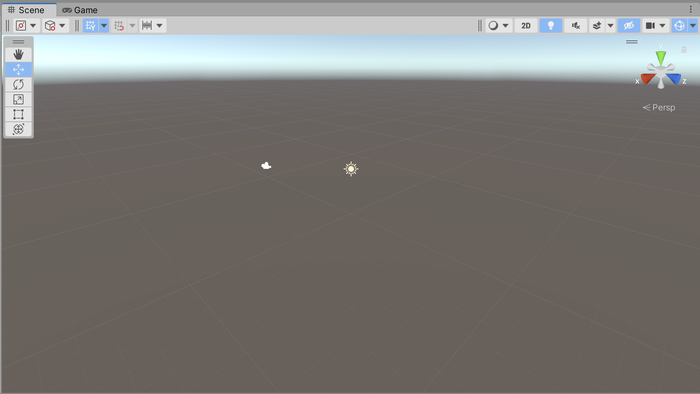
Figure 4: The Scene view
To the left of the Scene view, you'll find the Hierarchy. This panel represents all the game objects present in your current scene (which will be displayed visually in the Scene view). Each object has its place in this tree-like structure, making it easier to manage complex scenes with numerous objects. You can add GameObjects to your game's Scene by right-clicking in the empty space of the Hierarchy, and choosing what you might want to add to the Scene.
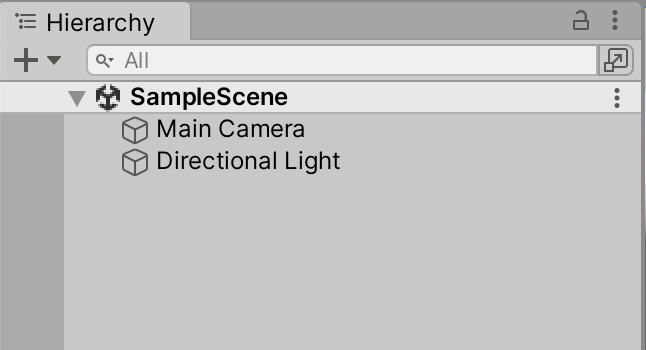
Figure 5: The Hierarchy
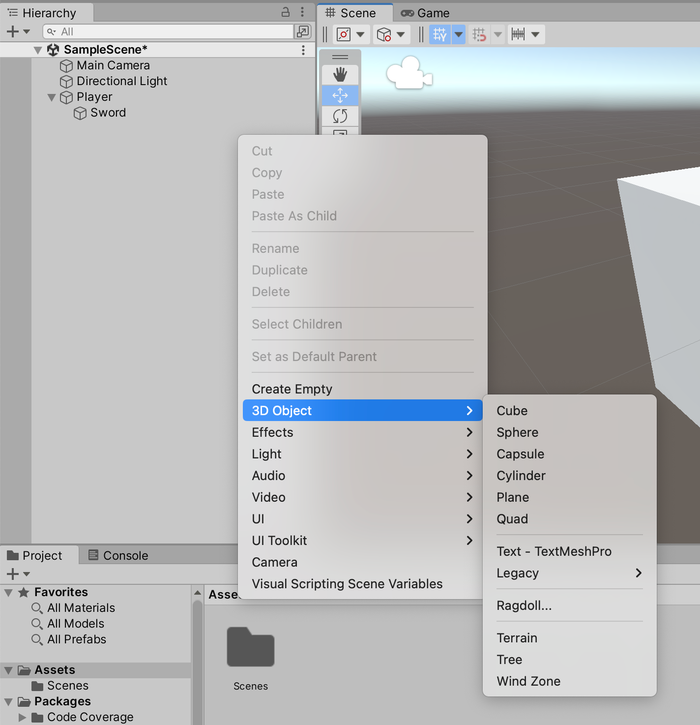
Figure 6: Creating a new GameObject which is added to the current Scene
On the right, the Inspector panel is the place where you'll modify the properties of your game objects. Select an object in your scene or Hierarchy, and the Inspector will display its components, each with its editable properties. You can add new components, such as scripts or physics attributes, from here. Below the Inspector panel, the Project view acts as a file explorer for your game. It hosts all the assets — such as scripts, models, textures, and audio files — that you can use in your project. You'll also find the Scene files you have saved in here.
Finally, there's the Game view tab. Clicking on this tab will give you a preview of what your game will look like when you hit play. Until moving components and logic are added, this will look much like the Scene view, but it's an invaluable tool to test and refine your game from a player's perspective.
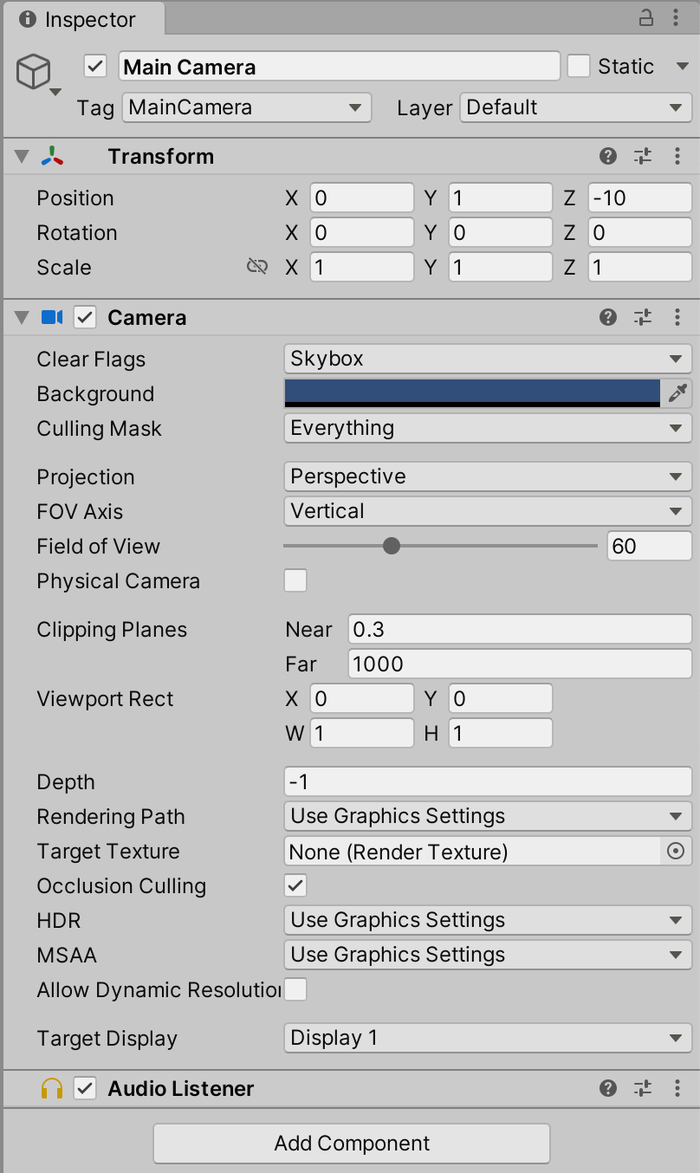
Figure 7: The Inspector (with the Main Camera selected)
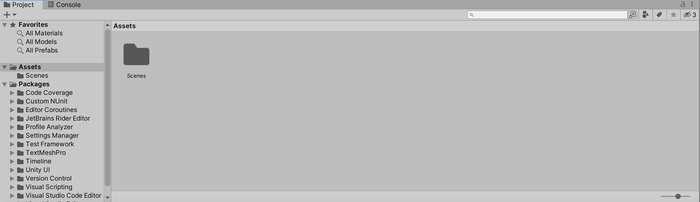
Figure 8: The Project view
Each of these components plays a crucial role in game development with Unity. While they might seem intimidating initially, remember that mastering Unity is less about memorizing each button's function and more about understanding these core components' roles, and how they all come together to help you build games.
As you start creating in Unity, you'll begin to build a natural understanding of the interface. Experiment, explore, and don't be afraid to make mistakes. Even the most experienced developers continually discover new tricks and methods in their Unity journey. Take a deep breath, shake off any initial sense of despair, and embrace the learning process. The Unity interface is your game development playground. It's time to play!
Next, let’s unpack how a game is assembled from pieces in Unity.
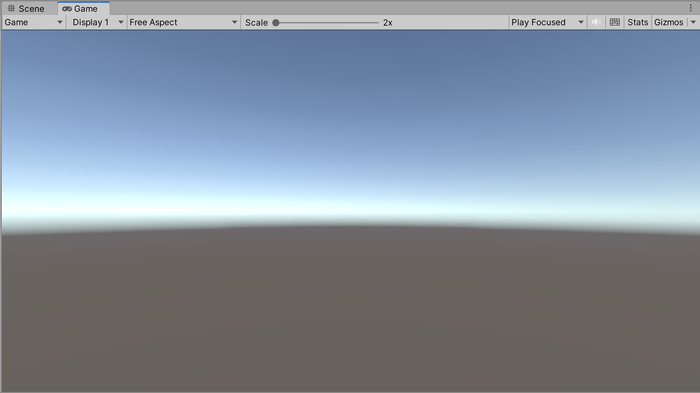
Figure 9: The Game view
Unity composition
In Unity, Scenes, and the GameObjects inside them, are the core building blocks of your game. GameObjects can represent anything, from characters and items to lights, cameras, or invisible containers.
Just as a Scene is an empty vessel for you to create a hierarchy of GameObjects within, GameObjects by themselves are also empty vessels: it's the Components attached to these GameObjects that give them life and functionality.
GameObjects always appear in the Hierarchy, and often have a "physical" presence in the Scene View. Each GameObject can have multiple Components, each defining a unique aspect of its behavior or appearance. The Components can be found by selecting a GameObject, and looking at the Inspector. For example, if we created a 3D Cube in the scene, shown here in the Scene View and in the Hierarchy, it would already have a whole lot of Components. These Components make it what it is.
Selecting the Cube in the Hierarchy then allows us to look at its Components in the Inspector.
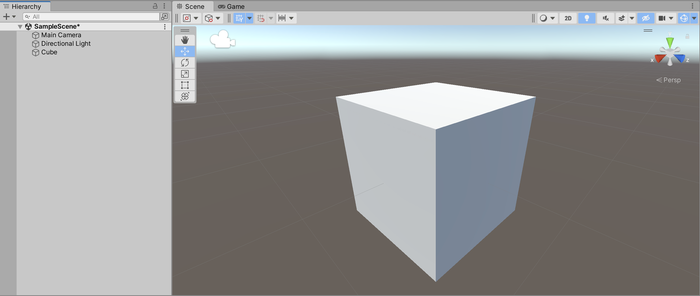
Figure 10: Our new, special 3D Cube GameObject
We can see that this humble cube has the following Components:
- A Transform, giving a position in 3D space;
- A Mesh Filter, provides a 3D mesh for the GameObject to use (in this case, a Cube);
- A Mesh Renderer, which describes the rendering properties of any mesh being rendered.
- A Box Collider, which defines how other objects that may interact with this GameObject may relate to it in a Physical (physics) simulation.
- A Material, which defines how the Mesh looks in the game world.
Going slightly further, we could consider a hypothetical player character in a 3D video game. At its core, it's going to be a GameObject. But what makes it a player character are the Components attached to it.
For example, a GameObject that is to be used as a 3D Player Character is likely to have the following components attached:
- A Transform: This is the only component that every GameObject has by default. It stores the object's position, rotation, and scale in the game world.
-A Mesh Filter and Mesh Renderer: This makes the GameObject visible. It uses a 3D model(called a mesh) and materials to define how the object looks.
- A Character Controller: A Character Controller is used when you need manual control over the
character's movements within the physical simulation.
- An Animator: This controls the animations of the character, allowing to animate when it walks, jumps, or perform actions.
- A Script: This is a script you write in C# (Unity's primary scripting language) to define the character's unique behaviors - for instance, responding to player inputs to move or interact with the game world. You can add Components to a GameObject by using the "Add Component" button at the bottom of its Inspector.
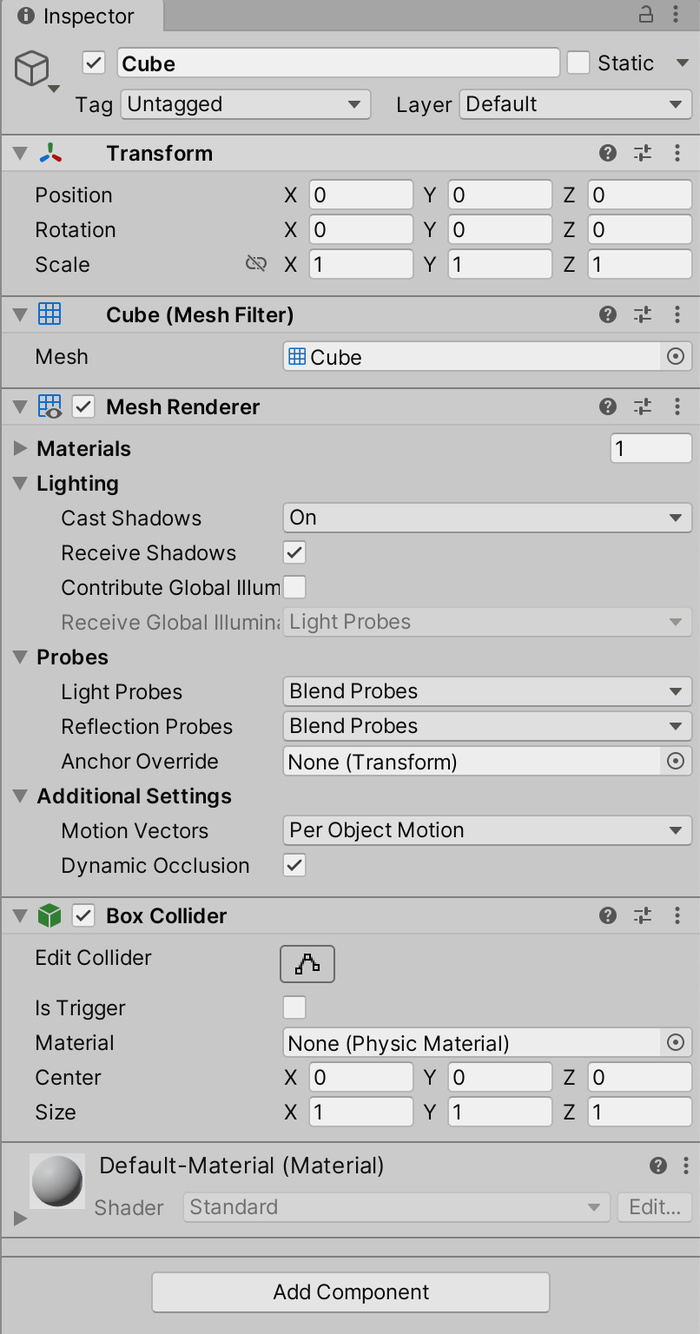
Figure 11: The Components and related properties of the 3D Cube GameObject
Hierarchies of GameObjects
GameObject hierarchies are another crucial concept in Unity. This is where GameObjects can become parents or children of other GameObjects, creating a tree-like structure.
For example, our player character might hold a sword. The sword would be a separate GameObject with its own Components (like a Transform and a Mesh Renderer). But, we would want the sword to move and rotate together with the player. To do this, we would make the Sword GameObject a child of the Player GameObject. Now, whenever the Player GameObject moves or rotates, the Sword GameObject follows suit.
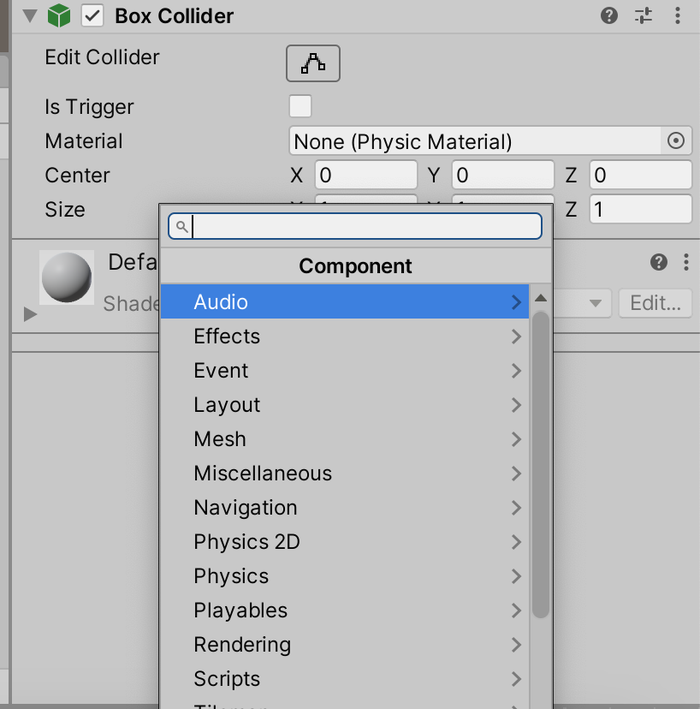
Figure 12: Adding a Component to a GameObject from the Inspector
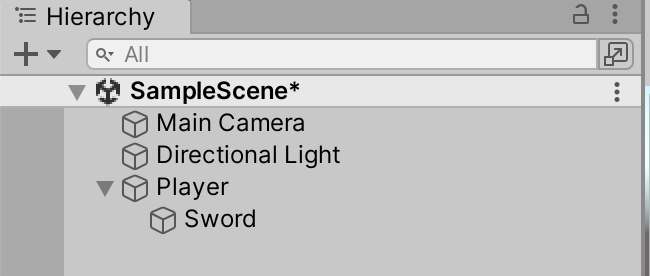
Figure 13: A Sword GameObject that is a child object of a Player GameObject
In more complex characters, you might have several layers of hierarchy. The player character might have GameObjects for the torso, head, arms, and legs—each with further GameObjects for aspects like fingers or facial features. Each of these could have their own Components defining their appearance and behavior. So, in Unity, GameObjects and their Components work together to create complex and interactive elements in your game. By understanding these structures and hierarchies, you can start to build a rich, interactive game world.
Where Scripting Fits In
At this point, you might be wondering where scripting and scripts fit in to all of this. Well, they're actually attached as Components on GameObjects. If we return to our humble Cube, and use the Add Component button to add a new Script to it, we can see how this works.
In this script, we're going to make our cube slowly rotate over time. To do this, let's create a script called "Rotate." Once the Script is added, you'll see it as an Component in the Inspector.
If you click it, you'll also see that it’s found in the Project pane as well, because it's an asset that is saved to disk in a persistent location. This saved version will be a C# file, so if you find it in your system's file explorer it will have the ".cs" file extension. Double-clicking that asset will allow us to edit it in our source code editor of choice. By default, Unity will install and use Visual Studio, but many users have preferred alternatives such as Visual Studio Code or others. This can be changed easily in Unity's preferences.
Inside the script, we've put the following code. We're not here to teach you the minutiae of programming for Unity, but let's step through what each part of this does...
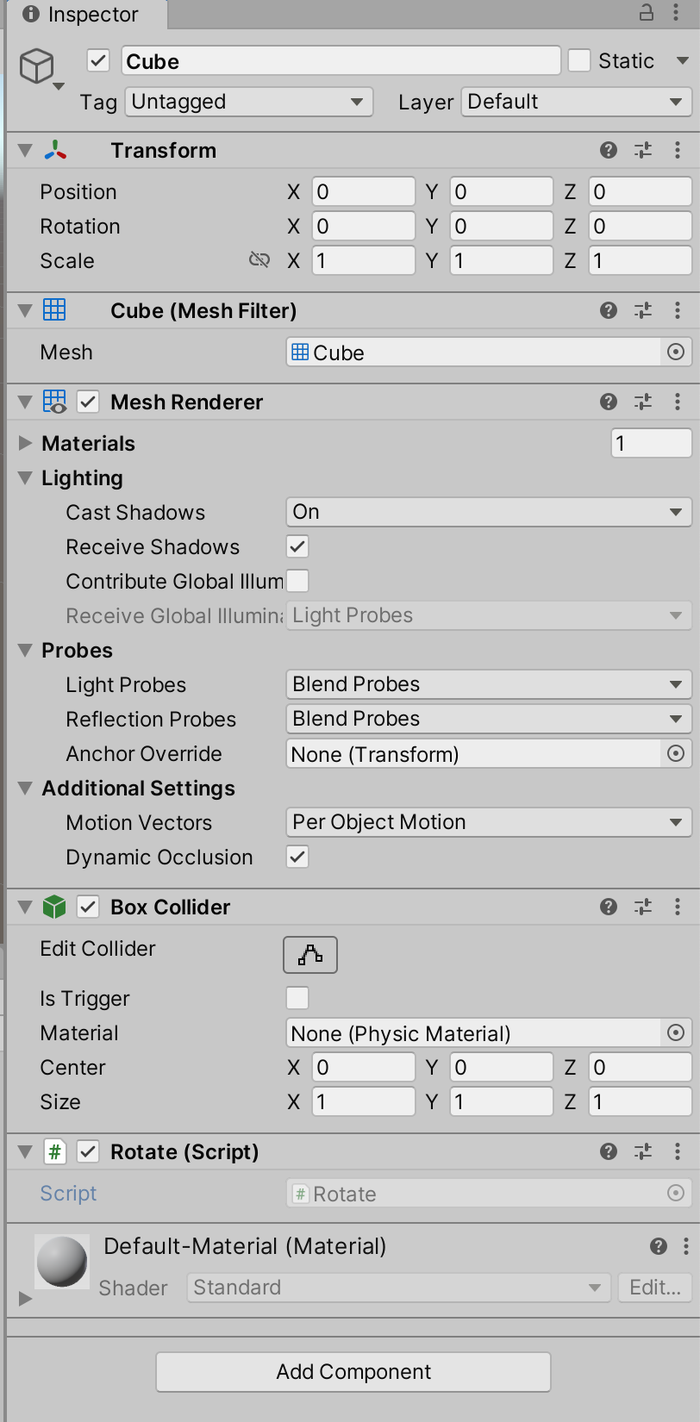
Figure 14: The Rotate component, as it appears in the inspector when the cube is selected.
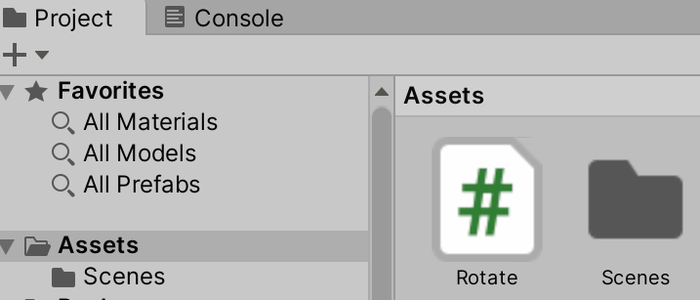
Figure 15: The Rotate script, as it appears in the project pane.
// import the UnityEngine namespace, giving access to the Unity-specific bits of C# using UnityEngine;
// define a class named Rotate, which inherits from the Unity base class MonoBehaviour
- (class name must match file name in Unity Scripts) public class Rotate : MonoBehaviour
{
// declares a floating point (decimal) number that will dictate speed of rotation // (by adding “f” this is a decimal, even though it doesn’t have a fractional part)
// by setting this to "public," it will become visible in the editor’s inspector
public float rotationSpeed = 10f;
// the Update() function is an inbuilt function that is called every frame
// so it is used to perform checks for state changes, or enact some ongoing change
void Update()
{
// here, it will rotate the object a tiny bit each frame by accessing the
// transform that we saw earlier in the editor's inspector,
// which defines the object’s position and rotation
transform.Rotate(Vector3.up * rotationSpeed * Time.deltaTime);
}
}
If you ran this, with this script attached to the humble Cube, the cube will slowly spin, and you could make it faster or slower by changing the variable in the editor, as it runs.
Conclusion
When you're working with Unity to build a game, you’re going to be jumping between the all the various views and areas we've just discussed: moving around your game world in the Scene View, arranging the Hierarchy of GameObjects, manipulating the Components attached to each GameObject (and adding new Components) in the Inspector, and using file-based assets (such as image textures and sound assets) from the Project View. Scripts in Unity are just another Component. A GameObject can have as many scripts as you need attached, and they can expose variables as needed to the editor. You'll then run your game, and switch to the Game View, which gives you the player experience of the game, and you'll go from there. And, little by little, you'll make something great!
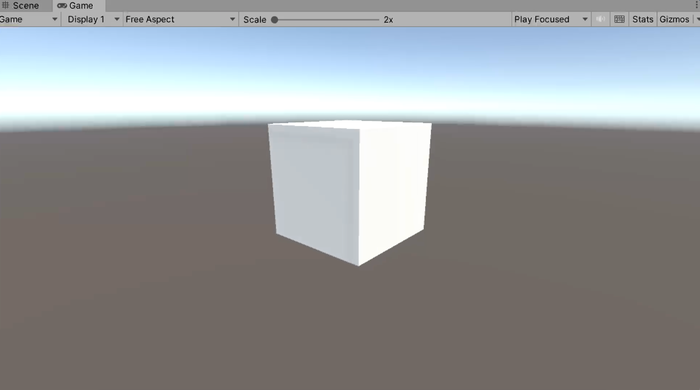
Figure 16: The humble rotating cube, with various values set for rotationSpeed.
Be sure to check out our other tutorial resources and lists for finding the best game development software for your needs.
About the Author(s)
You May Also Like